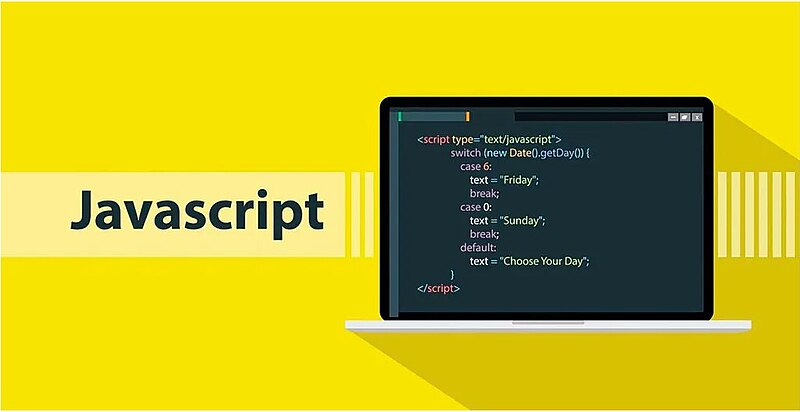
do you prefer to mimic jquery without loading its huge library, these approaches can replace js silly long selectors with more eye candy jquery like solutions
Approach | Usage | Pros | Cons |
---|---|---|---|
Basic Bindings | const $ = document.querySelector.bind(document); const $$ = document.querySelectorAll.bind(document); Example: Change background color: $('.class').style.background = "#BADA55"; Change inner HTML: $('#id').innerHTML = "<span>Cool beans!</span>"; | – Simple and clean syntax. – Directly utilizes native methods. | – Does not differentiate between single and multiple elements. – May lead to confusion if expecting an array. |
jQuery-like Functionality | const $ = (css, parent = document) => parent.querySelector(css); const $$ = (css, parent = document) => parent.querySelectorAll(css); Example: Select all images: $$('img'); Print image sources: $$('img').forEach(img => console.log(img.src)); | – Mimics jQuery’s syntax. – Converts NodeList to array for easier manipulation. | – Slightly more complex than basic bindings. |
Optimized ID Handling | const $ = (q, d = document) => /#\S+$/.test(q) ? d.querySelector(q) : Array.from(d.querySelectorAll(q)); Example: Get an element by ID: $('#id'); Get elements by class: $('.class'); | – Efficiently handles ID selectors. – Returns a single element for IDs and an array for others. | – More complex syntax may be less readable for beginners. |
Performance Consideration | const $ = (selector, base = document) => { let elements = base.querySelectorAll(selector); return (elements.length === 1) ? elements : elements; } Example: Single or multiple selection: const el = $('div'); | – Clear distinction between single and multiple selections. – Maintains performance by using native methods. | – Potential performance overhead due to additional checks. |
Legacy Support with Conflict Avoidance | const $q = document.querySelector.bind(document); const $qa = document.querySelectorAll.bind(document); Example: Use in legacy code: $q('#legacy-id'); | – Avoids conflicts with libraries like jQuery. – Useful in distributed teams or legacy codebases. | – Longer identifiers may reduce readability for those used to $ . |
Dynamic Selector Handling | const $ = s => document.querySelectorAll(s).length > 1 ? document.querySelectorAll(s) : document.querySelector(s); Example: Dynamic selection based on length: const result = $('div'); | – Combines functionality for single and multiple selectors in one call. | – Increases the number of calls, potentially impacting performance. |